When learning reactjs and redux I kept encountering build errors, bad practices and things that "Just didn't Work". I am going to attempt to list them all here in hopes that if I ever forget them I will have a reference point to work from.
Directory Layout
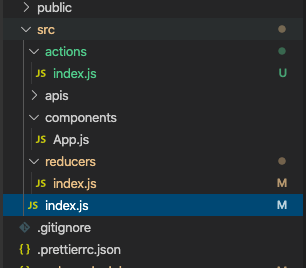
Reducers and Store setup
Coding react can be easy, integrating redux store can be confusing here is one instance I was getting an error I wasn't quite sure how to solve immediately.
./src/index.js
Attempted import error: './reducers' does not contain a default export (imported as 'reducers').
Fix
When setting up your provider in the ./src/index.js file you must put some "Dummy Data" into the reducers, then export it.
./src/Index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import { createStore } from 'redux';
import App from './components/App';
import reducers from './reducers';
const store = createStore(reducers);
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.querySelector('#root')
);
./reducers/index.js
import { combineReducers } from 'redux';
export default combineReducers({
replaceMe: () => 'DUMMY DATA'
});
Safe State updates for Reducers
Some ways of updating Arrays and Objects within redux.
Arrays
I will list some built in functions that do not modify the original state of the array, instead creates a completely new Array and leaves the original.
// Array
state = ["Hello", "World"];
// Add
[...state, "Travis"];
// Remove
state.filter(element => element !== "Hello");
// Update
state.map(element => element === "Travis"?"Jane" : element);
Objects
List of safe operations when working with objects within reducers. Again goal here is to keep the original object state unchanged.
Person = {"Jane Doe", number:300};
// Adding information
{...Person, age: 30};
// Removing a Property
_omit(Person, 'number');
// OR set it to "undefined" Not the best option IMO
{...Person, number: undefined}
//update a property
{...Person, name: 'Jonny Walker'};
ReactJS Datepicker
Setting up airbnb/react-dates with redux-form.
Step one, create a separate class based component.
import React, { PureComponent } from 'react';
import 'react-dates/initialize';
import { DateRangePicker } from 'react-dates';
import 'react-dates/lib/css/_datepicker.css';
class DateRangePickerField extends PureComponent {
state = { focusedInput: null };
handleFocusChange = (focusedInput) => this.setState({ focusedInput });
render() {
const {
meta: { error, touched },
input: {
value: { startDate = null, endDate = null },
onChange
}
} = this.props;
const { focusedInput = null } = this.state;
return (
<div>
<DateRangePicker
endDateId="endDate"
endDate={endDate}
endDatePlaceholderText="End Date"
focusedInput={focusedInput}
onDatesChange={onChange}
onFocusChange={this.handleFocusChange}
startDateId="startDate"
startDate={startDate}
startDatePlaceholderText="Start Date"
/>
{error && touched && <span>{error}</span>}
</div>
);
}
}
export default DateRangePickerField;
Step two, create a helper method to build the component renderDatePicker(), we pass a few props to help with styling and of course the datepicker functionality (input, meta).
renderDatePicker = ({ input, label, meta }) => {
return (
<div>
<label>{label}</label>
<DatePicker meta={meta} input={input} />
</div>
);
};
render() {
...
<Field
name="DatePicker"
component={this.renderDatePicker}
label="Enter Dates"
/>
...
}
Summary
I might be adding more to this post in the future if I feel its missing stuff. So far its been a fun challenge and experience.
Some of the biggest issues I encountered were the reducers and the actions side of redux, as well following best practices. For example, do I make dispatch calls outside the actions? or should I leave them exclusively in the action creators. I personally believe keeping them within the action creators will keep consistency, and anytime you have any questions like "Where did I make that dispatch call for 'X'?" Instead of breaking out your terminal and "grep -i dispatch . -R" Everything will be within your action creators.
I will be posting all the code to github.com/travism26
Comments