Transitioning from a monolithic to a microservice architecture with Docker and Kubernetes, integrating message brokers like Apache Kafka can significantly enhance the scalability and reliability of your applications. If you are looking to streamline communication between your microservices while maintaining high performance and fault tolerance, integrating Kafka into your Kubernetes environment is the way to go. In this guide, we will walk you through the process of integrating Kafka into your TypeScript microservice application running on Kubernetes.
Repo of entire application: https://github.com/travism26/microservice-examples
I am activity working on this repo and more functionality will be developed and I will write more in depth posts.
System Overview
Kafka is currently receiving messages from the auth service (Producer) and I added two consumers: User service to process newly created users and Event logger service to log all activity in the system. One can argue I need to add more logging across all my services, but to keep it simple I only added to auth service.
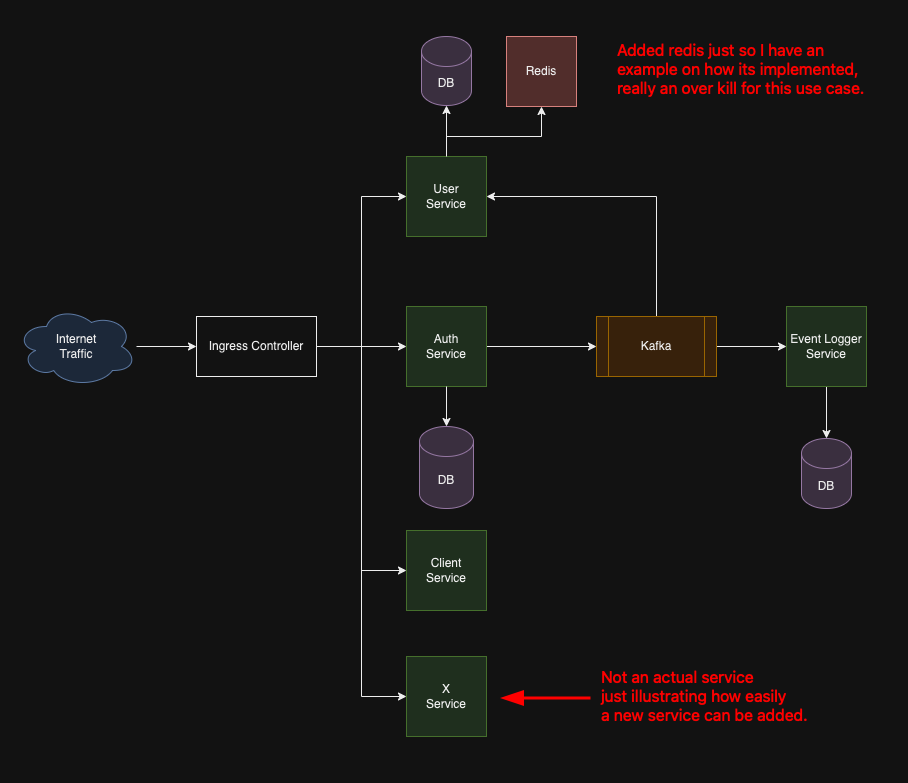
Setting Up the Environment
Before diving into the integration process, make sure you have a Kubernetes cluster up and running. Additionally, you will need a Kafka cluster deployed, which can be self-hosted on Kubernetes using tools like Strimzi Operator (This is my preferred approach).
Creating Abstract Class in TypeScript
Let's start by creating an abstract class in TypeScript that will serve as the blueprint for our Kafka consumer and producer implementations.
Implementing Kafka Consumer and Producer
Next, we will create concrete implementations of the Kafka consumer and producer by extending the KafkaClient abstract class.
Kafka Producer Implementation
Kafka Consumer Implementation
Managing Connections with Singleton Pattern
To ensure efficient resource utilization and connection management, we will implement a singleton pattern to manage Kafka client connections. Below is an example how I implemented my kafka wrapper that will be accessible across my entire application, I followed the mongoose singleton approach good article here explaining it. This kafka wrapper will be required on all your microservices, and might benefit putting this in your common library (Which I don't yet). Some implementation details I added two maps producers and consumers and their associated add function. Every time we add a producer / consumer we call the .connect() this ensures we are already connected, also all connections are managed within the singleton. Note might be great to add reconnect functionality.
Wiring up kafka into your application
Now that we have all the moving parts implemented, it's time to integrate the Kafka functionality into your TypeScript microservice application running on Kubernetes. Here’s how I implemented it, I created one producer and one consumer: SystemEventsPublisher, SystemEventsConsumer (Review my github repo for their implementation details) they extend the above Producer and Consumer abstractions. After they are implemented I wire everything together in my index.ts file located in the root dir.
index.ts Implementation
Conclusion
By integrating Kafka into your Kubernetes microservice application using TypeScript, you can unlock the full potential of event-driven architecture and build scalable, resilient systems. Whether you are processing real-time data streams, implementing event sourcing, or building a distributed messaging system, Kafka combined with Kubernetes offers a powerful solution. Implementing Kafka consumers and producers in TypeScript allows you to leverage type safety and maintainability while the singleton pattern aids in efficient resource management.
Now that you have a solid understanding of how to integrate Kafka into your Kubernetes microservices, start experimenting with different use cases and unleash the power of event-driven communication in your applications!
Remember, mastering Kafka and Kubernetes integration will undoubtedly propel your microservice architecture to new heights of performance and reliability.
Comentarios