I plan on building the classic parking garage interview question. The end goal is deploying this microservices architecture example to a managed kubernetes service. To make things slightly easier I will build each service using the Quarkus Framework, since the framework advertises kubernetes native it will reduce the overhead of integration. Also I would like to give some credit. I got some inspiration from a blog post: system design interview parking lot system I recommend reviewing this post as it goes into great detail on the design aspect, where I will go into the implementation and system architecture.
To start this off we need three microservices: Entry Service, Parking Service, and Spot allocation Service. This post will only include the user requesting a parking ticket, the pricing service and exit parking garage functionality has not yet been implemented.
System Architecture
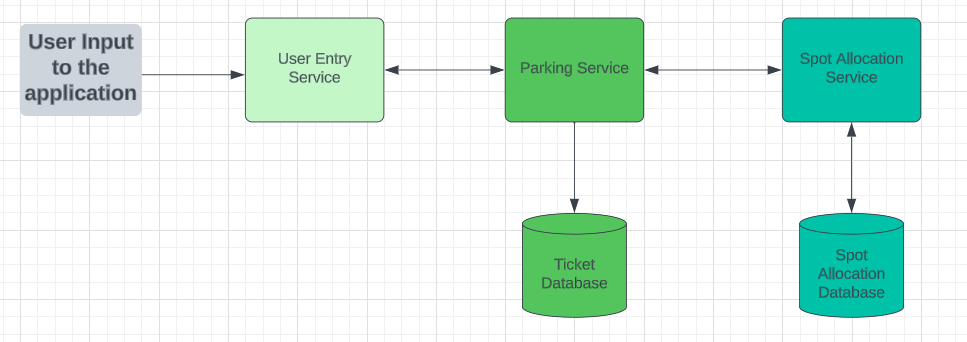
Implementation
I will not go into all the details of the code since most of the code is self explanatory. I will explain the details of each of the 3 microservices and their purpose. I will post the repository that contains all the code, as well as create a few more blog posts that will go into greater detail on different aspects of this implementation.
Entry Service
This service will take 1 parameter: vehicle json object with a license number and vehicle type. The main goal of this service is to take a request from the user and return a parking ticket. This parking lot will offer a few different types of parking spots, Motorcycles, Cars, Trucks and Larger vehicles like buses. The goal is to get the user input and output an assigned parking spot based on the user's vehicle.
Parking Service
This service will take 2 parameters: license number and vehicle type. It then sends a request off to the Service to assign a parking spot for the ticket. Once the ticket has been created, it gets persisted in the database and sent back to the Entry Service.
Spot Allocation Service
This service takes 1 parameter: vehicle type. It then searches the database for a free parking spot with that given type. There is room here for adding some parking spot optimizer to assign the closest parking spot near the exit, as well as adding a cache layer to speed up the database lookups. Once it gets the parking spot it returns two values: parking_spot, vehicle_size (size of vehicle compatible). Before returning the values to the parking service, it updates the database indicating that the parking spot is occupied.
Potential Bottlenecks
The current architecture is simple however there are some drawbacks. Currently the communication style is synchronous blocking, meaning we will have to wait until both the parking and spot allocation services complete before sending the ticket back to the Entry Service and finally the end user. Given the size of the system and users this should not be an issue. An alternative would be if there are any issues with the downstream services we assign a ticket with an empty parking spot and indicate to the ticket holder to go to the front desk to get assigned a parking spot. This approach can easily be accomplished with a fall back method if the request does not receive a response in a timely manner.
Persistence
The next layer to this is where we save all this data. I plan on using PostgreSQL, it is an open-source database management system (DBMS) that is known for its reliability, scalability, stability, and security. The only places that require persistence are Parking Service and Spot Allocation Service. The Entry service will act sort of like an API gateway, it will be the single point of entry and route the traffic to the appropriate services.
Communication Between Services
The communication between each service at the moment is synchronous blocking, meaning the Entry Service needs to wait for a response from some downstream process or in this case microservice to complete. Advantages to this approach are few, the code is easy to read and follow, for most programmers understand this type of communication. However there are more drawbacks to this approach. The entry service has to wait for the Parking Service which has to wait for the Spot Allocation Service, these open connections can cause resource issues on a larger scale. Knowing the potential issues of our current system this should not be an issue since only one user can request a ticket at a given time. Each system can also be isolated to a particular parking garage, and if we do want to add an additional feature online booking for example we can change the flow to asynchronous which I will cover later.
Communication Architecture Overview
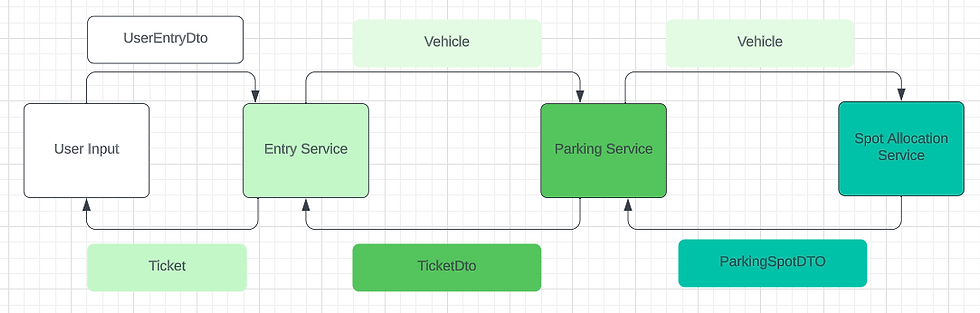
All endpoints produce and consume JSON, I annotated some of the attributes in the classes so that they are uniform throughout the entire application. The above diagram shows the input and output of each microservice with respect to their class. One thing to note here, is it appears we have shared models in the communication architecture. The name of the object Vehicle is similar,however, the bounded context of the Vehicle for each service is different. Parking service only cares about the license number where the Spot Allocation Service requires the Vehicle size. To keep things simple I make use of the vehicle class name and encapsulate everything within it.
Example of Vehicle Class

I applied the JsonProperty annotation to make the input and output of both consuming and producing services uniform regardless of the variable name (Although the names are exactly the same in each service).
Endpoint implementation
I will go over each endpoint showing the api calls made and where the objects are returned for each service. For the most part, the code is self explanatory, the confusing parts might be around the communication between services.
Entry Service
The entry service is the first point of user contact, generally this is where we might want to add some extra validation around user input. This precaution is to prevent hackers from sending malicious payloads and gaining unauthorized access. I will not add any checks to keep things simple, but it's recommended that you always sanitize user input.
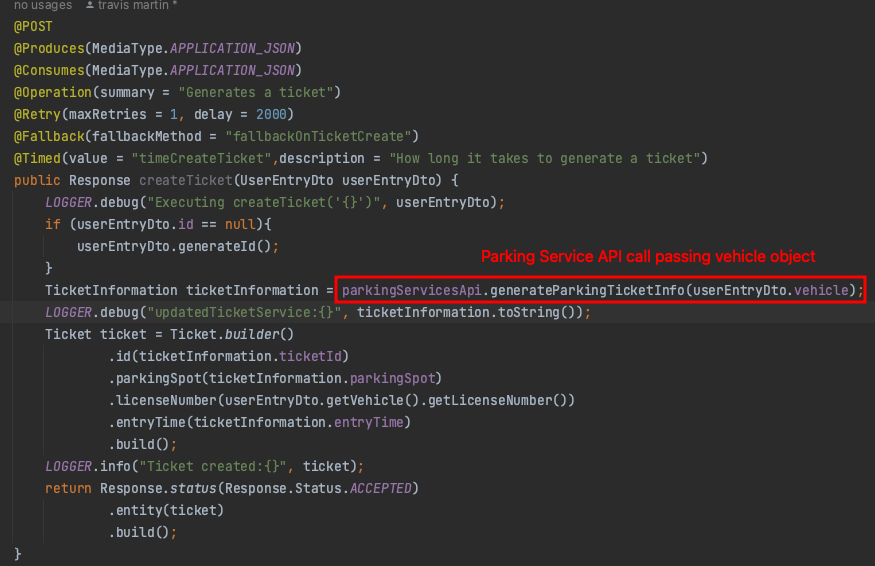
UserEntryService Endpoint implementation
I highlighted where I make an api call to the parking service passing in the vehicle object, this will save all the information in the ticketInformation variable. If there are any issues downstream, we will call the fall back method: fallbackOnTicketCreate this will generate a ticket that will indicate to the user to head to the customer service desk.
Parking Service
The parking service is simply a middle man. It takes a request made from the entry service, and then sends a request to the spot allocation service. Once the spot allocation service completes it then saves the ticket to the database and returns the ticketDto to the entry service. The persistence layer requires some extra functionality to interact with the database. I will write a separate post that will go into greater detail since this is just an overview.
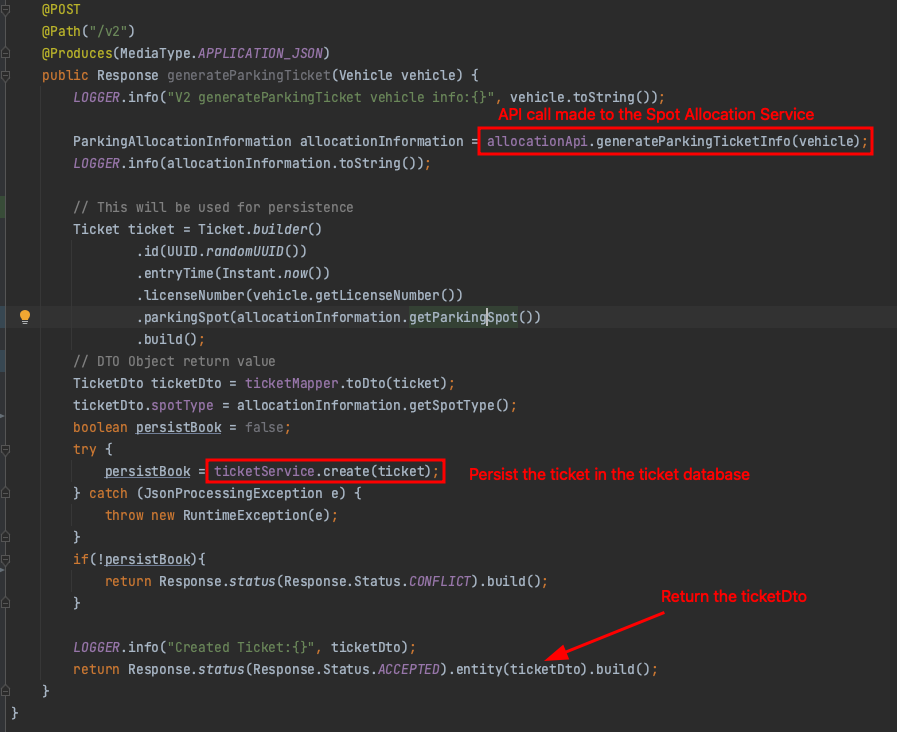
ParkingService Endpoint implementation
Spot Allocation Service
This is the last service that contains the list of unoccupied parking spots with their corresponding size. The responsibility of this service is to take a request with a vehicle size and respond with a parking spot that the vehicle will fit in. Note I did not add any fallback methods to the previous two endpoints. Plan was to add it to the first endpoint showing how it can be accomplished. I opted to skip these two services but just want to note they should be added eventually.
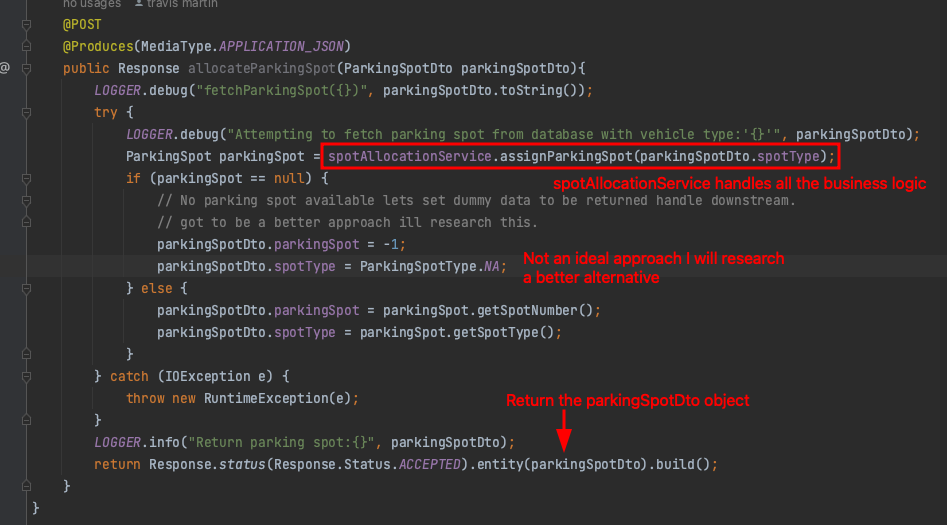
SpotAllocationService Endpoint implementation
Execution
Now that I explained how everything works and how each of the services communicate with each other, I will run them locally and demonstrate how they work.
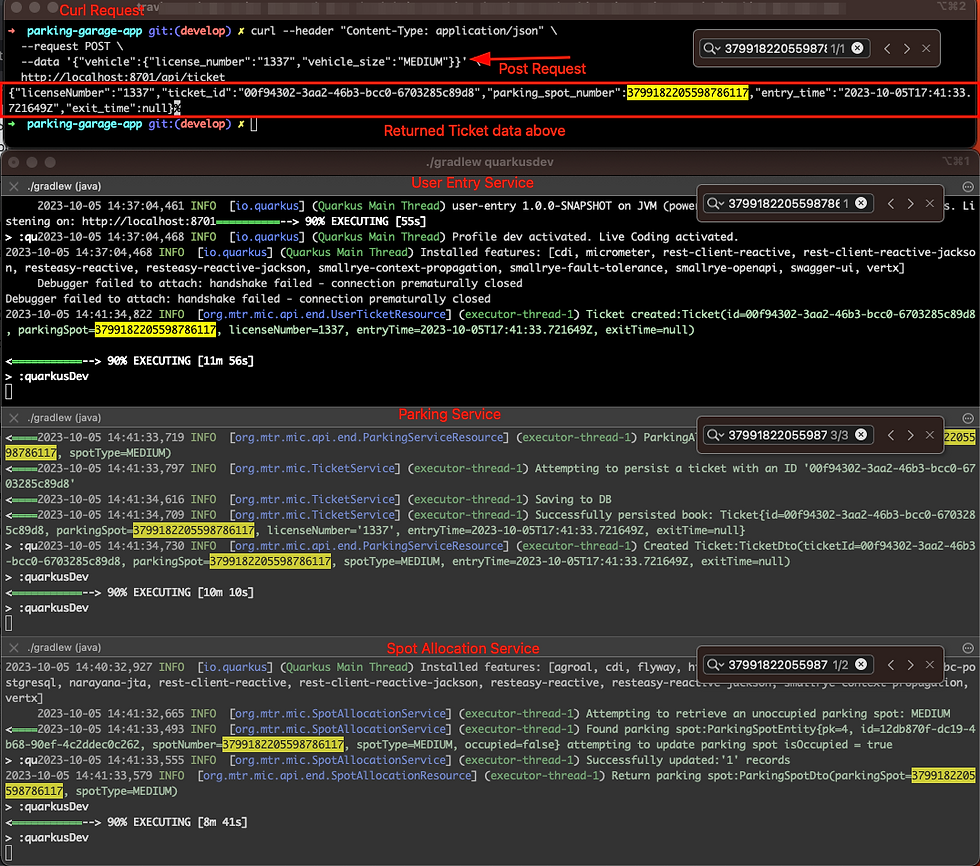
Execution of 3 services and requesting terminal
The above image shows 3 services running independently from one another, and the top most terminal is used to make a POST request using curl. I started the flow of information going from top to bottom. Starting with the User Entry Service, requests a ticket from the Parking Service, which requests a parking spot from the Spot Allocation Service. Once the allocation service assigns a spot it sends it back to the Parking Service, which in turn creates a ticket and returns it to the User Entry Service. I also highlighted the parking spot returned to the user on all three services to show the flow of data throughout the system.
Summary
This small application was not difficult to build. I made use of a lot of features provided by Quarkus. I mostly wanted to demonstrate the microservice communication while utilizing persistence on each service. I plan on adding more to this repository, expanding it and deploying it on a managed kubernetes service. If you found this useful please share this post or if you want me to build something else please put your suggestions in the comments.
Learning Resources
Some of the ideas I am applying here are taken from the course offered at bytebytego.com I highly recommend this site for learning about system design at a high level. Again I would encourage readers to visit this blog post about this design: system design interview parking lot system.
The code for this application can be found on my github page (github.com/travism26)
Final Comments
This being an overview of the application architecture I plan on diving deeper into a few other aspects of this application such as: Testing, Persistence, and deploying this to a kubernetes cluster. If you made it to the end, thank you for your time. If you see any errors or can suggest any improvements, please post them in the comments or open an issue in github.
Comments